REST (Representational State Transfer) on arhitektuurimudel, mida kasutatakse veebiteenuste loomisel ja rakenduste vahelise suhtluse lihtsustamiseks
import "./styles.css";
// Initialize variables to hold profile information
let profileName = "";
let profileId = "";
let profileLink = "";
let profileRepos = "";
let profilePicture = "";
// Asynchronous function to fetch GitHub profile information
async function fetchProfile(username) {
try {
// Fetch data from GitHub API for the given username
const response = await fetch(`https://api.github.com/users/${username}`);
// Parse the JSON response
const data = await response.json();
// Extract profile information from the response data
profileName = data.login;
profileId = data.id;
profileLink = data.html_url;
profileRepos = data.public_repos;
profilePicture = data.avatar_url;
// Render the profile information on the page
renderPage();
} catch (error) {
// Log any errors that occur during the fetch operation
console.error("Error fetching profile:", error);
}
}
// Function to render the profile information on the page
function renderPage() {
document.getElementById("app").innerHTML = `
<div>
<h1>Github profile viewer</h1>
<p>Please enter profile name: </p>
<input id="username-input" />
<div class="content">
<h1 id="name">Name: ${profileName}</h1>
<p id="id">Id: ${profileId}</p>
<p id="reports">Public repositories: ${profileRepos}</p>
<p id="profile-url">
Link: <a href="${profileLink}" target="_blank">/users/${profileName}</a>
</p>
<div id="profile-avatar">
<img src="${profilePicture}" alt="${profileName} wait for profile picture...." style="width: 100px; height: 100px; border-radius: 60%;" />
</div>
</div>
</div>
`;
// Add an event listener to the input field to handle 'Enter' key press
document
.getElementById("username-input")
.addEventListener("keyup", function (event) {
// Check if the 'Enter' key was pressed
if (event.key === "Enter") {
// Get the value from the input field
const username = event.target.value;
// Fetch the profile data for the entered username
fetchProfile(username);
}
});
}
// Initial call to render the page with default content
renderPage();
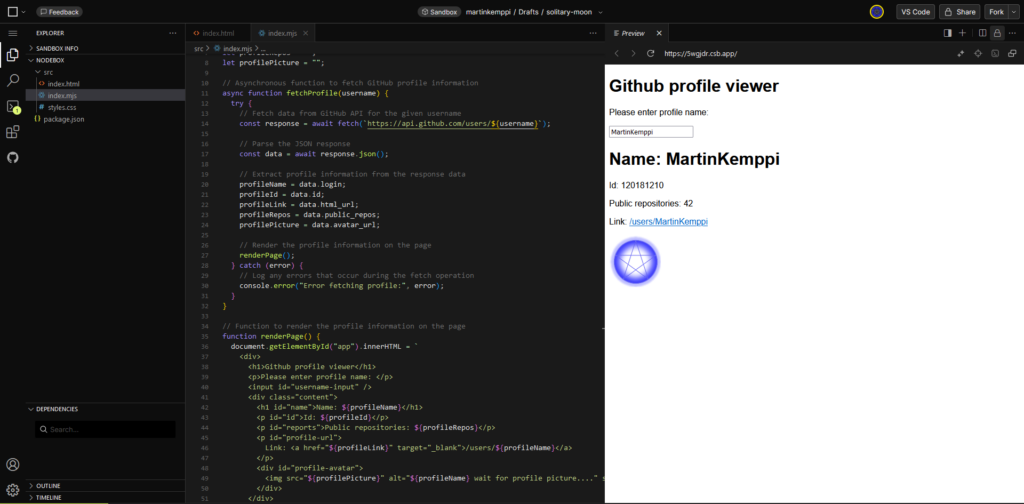